Recently I’ve decided to move away from Storyboards and XIBs, in favor of the clarity coded views give.
Combined with Anchorage, this gives for some nice and readable layouts, and doesn’t produce those near-unresolvable conflicts if I have to fixup
and rebase
.
However, I started to feel as if I was fighting against the framework. Turns out I was π±
UIViews and me
My lay-outing process for coded views usually consisted of something like
let customView = MyCustomView()
someParentView.addSubview(customView)
Whether this’d be in order to reuse a component I had written, an adjustment to existing views, … this pattern recurred a lot. And although I have tried laying out my views in code before, that was always accompanied with a lot of pain due to a lot of effort to get it rightπ
Seemed to me that the method .loadView()
would be the logical and correct way to start doing this, right?
Turned out it is, but there’s one important caveat!
You’re doing it wrong
Now, what’s your reflex when you see this signature?
override func loadView() {
}
Mine was to call super.loadView()
, because… it feels right to call the super
method, right?
Well, the Apple docs kindly told me otherwise!
You can override this method in order to create your views manually. If you choose to do so, assign the root view of your view hierarchy to the view property. The views you create should be unique instances and should not be shared with any other view controller object. Your custom implementation of this method should not call super.
Apple being like: βοΈsuper.loadView()
βοΈ
You know that feeling that you get when everything suddenly falls in place?
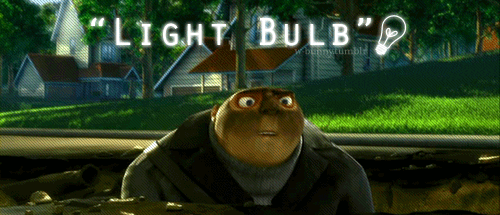
Now the only thing left to do was finding the correct autoResizingMasks
, and suddenly everything started to work the way I expected it to Β―\_(γ)_/Β―
Doing it right
Here’s the gist (π₯):
Make sure you don’t set translatesAutoresizingMaskIntoConstraints
to false
on the outer view. Leaving this setting to its default value makes sure iOS resizes our top-level view automatically.
Now I can just create my view, give it a default frame, and the view’ll resize nicely, without any added pain or bloat. π